Getting Started with Docker
Why Use Docker?
Docker is a tool that is used to automate the deployment of applications in lightweight containers so that applications can work efficiently and similarly in different environments.
Basic vocabulary
An image is the setup of a virtual computer. It is a package or template just like a virtual machine (VM) template in the virtualization world.
A container is a running instance of an image. It is a software package that consists of all the dependencies required to run an application which includes code, run-time, system tools, system libraries, and settings. It is isolated and has its own environment and set of processors.
Overview
Suppose you are working on a project and you want to send your code to a colleague for testing, your colleague runs the exact code but gets slightly different results. This inconsistency could arise from various factors such as a different operating system and different library or dependency versions among other issues. Docker is trying to solve issues like that.
An example of how Docker is trying to resolve issues such as the “it works on my machine syndrome” can can illustrated by drawing a parallel with Git. We write code, push it using Git to a remote repository such as GitHub and another person who is somewhere across the world can pull it and have the same copy of code. Docker similarly, allows you to build an environment or infrastructure, push it to a docker registry such as DockerHub and a person across the world who has Docker on their machine, can pull down your docker image and have it run the same way it runs on your computer. This consistency in application behavior is achievable through the use of Docker containers, which allow software applications and processes to run in isolation.
According to a survey by RightScale (a cloud management company), Docker has become one of the most popular DevOps tools for large and small to medium enterprises. The section below discusses Docker’s main benefits which could possibly explain why it has gained DevOps traction in the software development industry at such a phenomenal rate.
Docker container benefits
Resolve dependency issues through isolation. When setting up a development environment, you will need to ensure that your software package is compatible with your version of the OS. Secondly, you will need to check if the package is compatible with the libraries and dependencies on the OS. This architecture changes over time and whenever it changes we have to go through the same process of checking compatibility between these components and the underlying infrastructure.
Challenges that may arise as a result of different services requiring different versions of the same software package are mitigated by Docker’s concept of container isolation; dependencies or settings within a container will not affect any installations or configurations on any other containers that may be running or on your computer. In Docker you can have Apache server in one container, MySQL within another and PHP in yet another all wrapped up in one host container running some version of Linux. These containers are completely isolated from one another and contain their own software, libraries and configuration files. The containers can, however, communicate with each other using well-defined channels.
Reduced set-up time for new developers. Every time a new developer joins a team, there are usually difficulties and /or delays when setting up their local development environment. The developer usually has to spend a lot of time reading installation instructions followed by running lots of commands to finally set up the development environment. During this set up they have to make sure that they are using the right OS, right software packages and each developer has to set that up each time. With Docker the developer or system administrator does not have to go through lots of set up instructions and run a lot of commands; if they already have Docker on their machine they just need to pull down the Docker image and start it up by issuing a simple command and they can almost immediately start to interact with their application.
Environment Management: Docker containers makes it easy to maintain code on different environments. Each of the testing, development and production environments can have their own separate containers on the same node and easily deploy to each other.
Reproducibility. One developer can be comfortable with using one OS and the other another, so we cannot guarantee that the app we are building will run the same in different environments. This issue is addressed by Docker containers which guarantee same application behavior across different environments. Docker containers also make it easier to reproduce bugs on production since the same container/environment on production can be run on your local machine in the same way giving the exact results.
Continuous Integration: Docker integrates well as part of continuous integration pipelines with other tools such as Git, Jenkins and Ansible. For example, whenever an update is made to your source code in a git repository , Jenkins triggers a build and Ansible uses the file resulting from the build to create the new version of a docker image, tag it and push it to DockerHub by executing Docker commands in the playbook, then finally it deploys to production.
In conclusion, like all new technologies Docker is still being refined and it has its limitations and it is also import to note that it cannot be used on all projects. Enough research should be conducted to identify the projects in which the wealth of docker benefits can be leveraged to optimize the development, building and shipping of an organizations software applications.
Setting up a simple Nginx Web Server in a Docker Container.
Installing Docker Desktop on a Mac
Main System requirements.
- Mac hardware should be a 2010 model or newer.
- Mac Os must be version 10.13 or newer
Installation
- Download Docker
- Double click the “Docker.dmg” file you just downloaded, to start docker installation.
- Once the installation completes and Docker is started you should be able to see the Docker whale in the top status bar
Installing Docker on Windows 10
Main System requirements
- Windows 64-bit
- Windows Pro, Education, Enterprise, 1511 November update, Build 10586 or later
Installation
- Download Docker
- Double click the InstallDocker.msi to start the installer
- Follow the installation wizard to proceed with the install
- Click Finish to launch docker
- Docker will start automatically
Installing docker on Linux Ubuntu
To install Docker go here
Running Docker
Open the terminal and try out the commands below:
docker version
docker ps
Lists all available containers
docker image ls
Lists all the images
docker run hello-world
Verifies if your docker installation is running as expected.
Setting up Simple Nginx server in a Docker Container in a few steps:
The great thing about Docker is there are tens of official images that you can simply download and are ready to be used. In this case you will not need to create any Dockerfile, for example you can download and use a lamp stack, Apache web server, Nginx web server images out of the box.
In the following example, we will create a NGINX container named simple-nginx-container. We will pull an image called nginx:latest from Docker Hub.
Steps
1. Create a directory at your preferred location.
2. cd into this directory.
3. Pull the latest nginx docker image from dockerhub by running the command below:
sudo docker pull nginx:latest
4. Run the command below :
sudo docker run -d --name simple-ngix-container -p 4000:80 -v /Path/to/your/local/directory/:/usr/share/nginx/html/ nginx:latest
The command above creates a docker container “simple-nginx-container” from the image “nginx:latest”. We will have the request made on our public IP address on port 4000 to be redirected to port 80 on our container. We are mapping /Path/to/your/local/directory/ (directory you created in step 1) to /usr/share/nginx/html/, we do this to server content from /Path/to/your/local/directory/ instead of serving content directly from the container. Ensure that you don’t leave out the forward slashes at the end of the directories.
5. Create an index.html file inside /Path/to/your/local/directory/
by running the command:
vi index.html
6. Paste the code below:
<!DOCTYPE html>
<!DOCTYPE html>
<html>
<head>
<title>Welcome to Docker!</title>
<style>
body {
width: 35em;
margin: 0 auto;
font-family: Tahoma, Verdana, Arial, sans-serif;
}
</style>
</head>
<body>
<h1>Welcome to Docker!</h1>
<p>This is a simple NGINX Web Server in a Docker Container</p>
<p>Learn more at
<a href="http://thedevopsevangelist.com/">thedevopsevangelist.com</a>.
</p>
<p><em>Thank you for completing the exercise.</em></p>
</body>
</html>
Open your browser and point to 127.0.0.1:4000/index.html you should see:
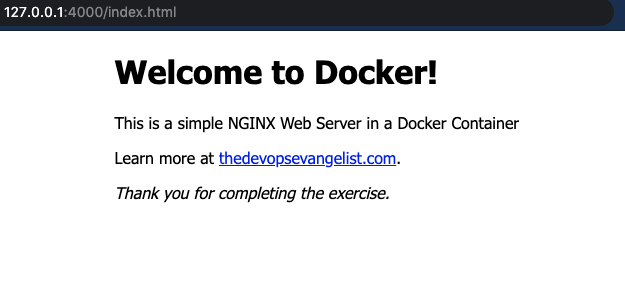
If you wish to stop the container run the command below:
sudo docker stop simple-ngix-container
To remove it run:
sudo docker rm simple-ngix-container
To complete cleaning up you will need to delete the image used in the container.
sudo docker image remove nginx:latest
To confirm if the containers have been deleted run:
Sudo docker ps
To confirm if the image have been deleted list all available images by running the command below:
Sudo docker images -a